Doubly Circular Linked List
- Code Craze
- Mar 14, 2023
- 1 min read
A doubly circular linked list is a type of linked list where each node has both a "next" and a "previous" pointer, allowing for traversal in both directions. Additionally, the last node in the list points back to the first node, forming a circle.
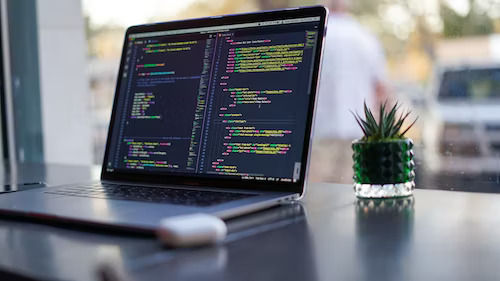
Kommentare