- Code Craze
- Jan 20, 2023
- 1 min read
Updated: Jan 22, 2023
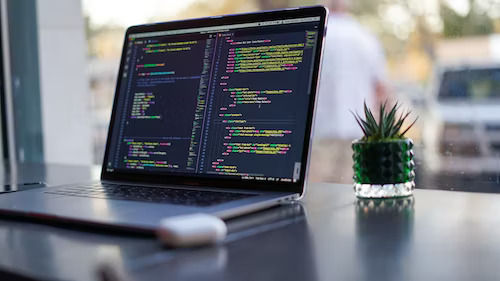
#include <stdio.h>
void main() {
int num1, num2,
float avg;
printf("Enter the first number: ");
scanf("%d", &num1); // Read the first number from the user
printf("Enter the second number: ");
scanf("%d", &num2); // Read the second number from the user
avg = (num1 + num2)/2; // Calculate the avg
printf("The average of %d and %d is %f\n", num1, num2, avg); // Print the result}
Here's a brief explanation of the code:
The first line #include <stdio.h> is a preprocessor directive that tells the compiler to include the standard input/output library. This library contains functions like printf and scanf that are used to read input and print output.
int main() is the main function where the program starts executing.
int num1, num2, avg; declares three variables: num1 and num2 to store the two numbers and avg to store the result.
printf("Enter the first number: "); prints the message "Enter the first number: " on the screen, asking the user to enter the first number.
scanf("%d", &num1); reads an integer from the user and stores it in the variable num1. The & operator is used to pass the address of the variable to the function, so that it can store the input in that location.
The same steps are repeated for the second number.
avg = (num1 + num2)/2; calculates the average of num1 and num2 and stores it in the variable avg.
printf("The average of %d and %d is %d\n", num1, num2, avg); prints the result on the screen. The %d, %f is a placeholder for an integer and float.
You can run this program on a C compiler.