- Code Craze
- Feb 12, 2023
- 1 min read
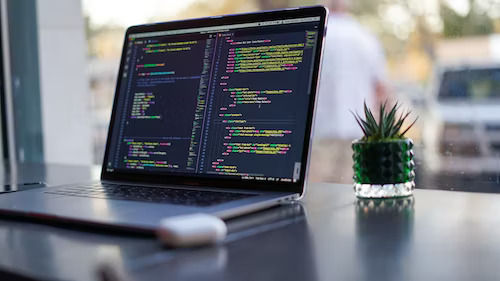
Integer: a whole number, either positive or negative (e.g. 42, -100).
Float: a real number, with a decimal point (e.g. 3.14, -0.01).
String: a sequence of characters (e.g. "hello", "goodbye").
Boolean: a value that can either be True or False.
Array: a collection of values of the same data type.
Dictionary: a collection of key-value pairs, where each key is associated with a value.
Object: a user-defined data structure that can contain data and functions.